Python Package gr¶
Installation¶
GR is available on PyPI and we recommend installing it with pip:
pip install gr
This will automatically install both the GR runtime and the Python wrapper. You may need to install some additional dependencies on Linux:
- Debian:
apt install libxt6 libxrender1 libxext6 libgl1-mesa-glx libqt6widgets6
- Ubuntu:
apt install libxt6 libxrender1 libxext6 libglx-mesa0 libqt6widgets6
- CentOS / Fedora / Rocky Linux:
yum install libXt libXrender libXext mesa-libGL qt6-qtbase-gui
- openSUSE:
zypper install libXt6 libXrender1 libXext6 Mesa-libGL1 libQt6Widgets6
- Arch Linux:
pacman -S mesa qt6-base
Note: Depending on the Linux flavor you are using, the package names may differ from the ones mentioned here. This particularly applies to the Qt versions used.
- On FreeBSD make sure to install the these packages:
pkg install libXt libXrender libXext mesa-libs qt6
For information on building the GR runtime yourself, see Building the GR Runtime.
Docker and other headless Linux systems¶
GR does not rely on X11 for its non-interactive output formats, so you will not need the dependencies listed above on a headless system.
GR3 uses GLX for OpenGL context creation, which requires a connection to an X server. If you are using a headless sytem, e.g. a Docker container, you can use Xvfb or similar tools to start an X server that can be used by GR3, although it may only provide software rendering.
Getting Started¶
After installing GR, you can try it out by creating a simple plot:
from gr.pygr import mlab
mlab.plot(range(100), lambda x: x**2)
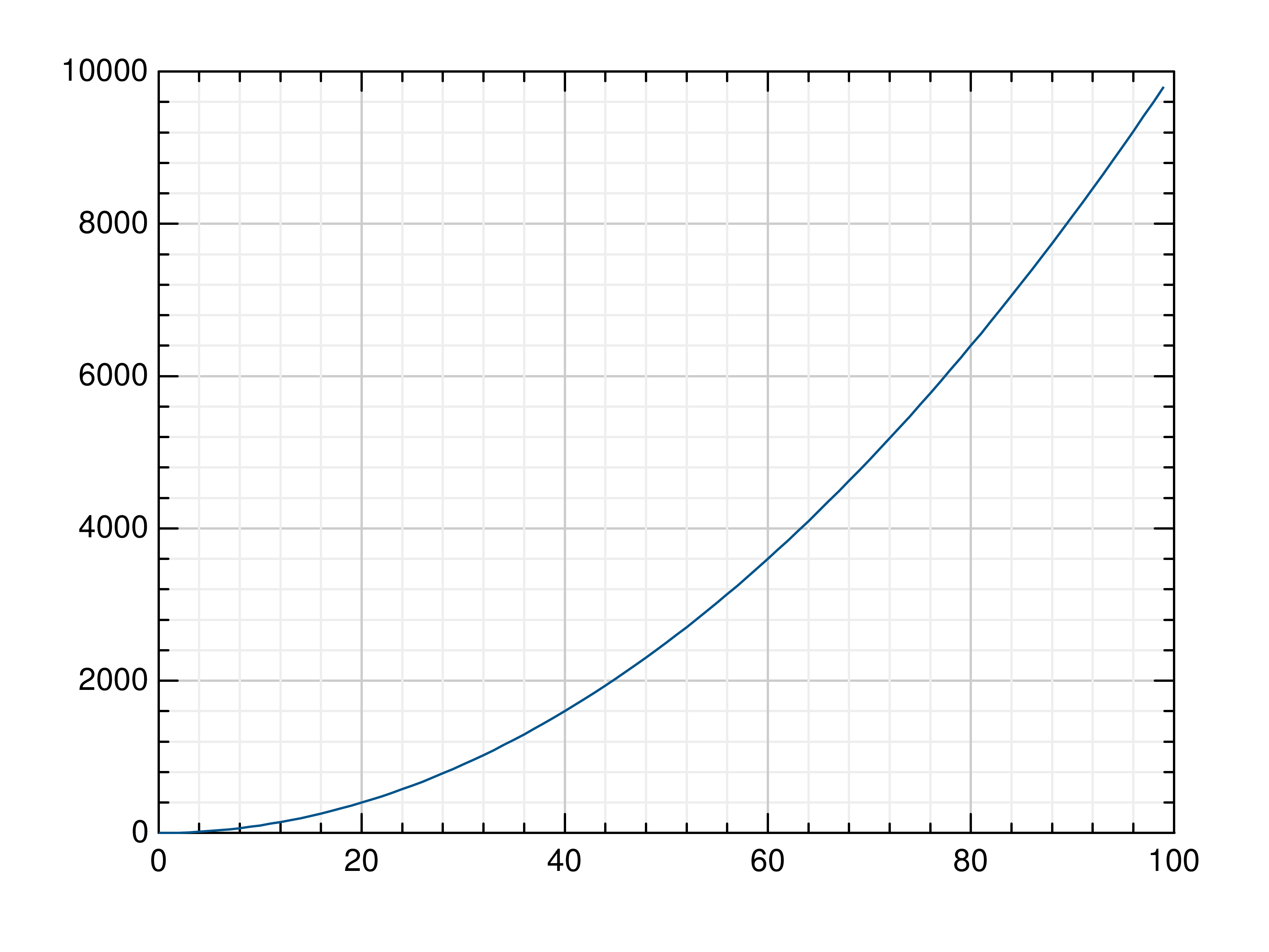
Tutorials¶
You can find several tutorials on using GR in the Tutorials section.
Examples¶
You can find a collection of Python scripts using GR in the Examples section.
API Reference¶
The Python API for GR consists of:
- gr Reference
- Output Functions
- Attribute Functions
setlinetype()
setlinewidth()
setlinecolorind()
setmarkertype()
inqmarkertype()
setmarkersize()
inqmarkersize()
setmarkercolorind()
inqmarkercolorind()
settextfontprec()
setcharexpan()
settextcolorind()
setcharheight()
setcharup()
settextpath()
settextalign()
setfillintstyle()
setfillstyle()
setfillcolorind()
setcolorrep()
setcolormap()
setcolormapfromrgb()
setarrowstyle()
setshadow()
settransparency()
setresamplemethod()
inqresamplemethod()
setbordercolorind()
inqbordercolorind()
setborderwidth()
inqborderwidth()
- Transformation Functions
setscale()
inqscale()
setwindow()
inqwindow()
setviewport()
selntran()
setclip()
setwswindow()
setwsviewport()
setspace()
inqspace()
setcoordxform()
setprojectiontype()
inqprojectiontype()
setperspectiveprojection()
inqperspectiveprojection()
setorthographicprojection()
inqorthographicprojection()
settransformationparameters()
inqtransformationparameters()
camerainteraction()
setwindow3d()
inqwindow3d()
setscalefactors3d()
inqscalefactors3d()
- Control Functions
- gr.pygr Reference
CoordConverter
Coords2D
Coords2DList
Coords3D
Coords3DList
DeviceCoordConverter
ErrorBar
GridCoords3D
Plot
Plot.addAxes()
Plot.addROI()
Plot.autoscale
Plot.drawGR()
Plot.getAxes()
Plot.getROI()
Plot.isLegendEnabled()
Plot.logXinDomain()
Plot.logYinDomain()
Plot.offsetXLabel
Plot.offsetYLabel
Plot.pan()
Plot.pick()
Plot.reset()
Plot.select()
Plot.setGrid()
Plot.setLegend()
Plot.setLegendWidth()
Plot.setLogX()
Plot.setLogY()
Plot.sizex
Plot.sizey
Plot.subTitle
Plot.title
Plot.viewport
Plot.xlabel
Plot.ylabel
Plot.zoom()
PlotAxes
PlotAxes.COORDLIST_CLASS
PlotAxes.COUNT
PlotAxes.OPTION
PlotAxes.SCALE_X
PlotAxes.SCALE_Y
PlotAxes.addCurves()
PlotAxes.autoscale
PlotAxes.backgroundColor
PlotAxes.curveDataChanged()
PlotAxes.curveVisibilityChanged()
PlotAxes.doAutoScale()
PlotAxes.drawGR()
PlotAxes.getBoundingBox()
PlotAxes.getCurves()
PlotAxes.getId()
PlotAxes.getVisibleCurves()
PlotAxes.getWindow()
PlotAxes.isGridEnabled()
PlotAxes.isReset()
PlotAxes.isXDrawingEnabled()
PlotAxes.isXLogDomain()
PlotAxes.isYDrawingEnabled()
PlotAxes.isYLogDomain()
PlotAxes.majorx
PlotAxes.majory
PlotAxes.plot()
PlotAxes.reset()
PlotAxes.scale
PlotAxes.scaleWindow()
PlotAxes.setGrid()
PlotAxes.setLogX()
PlotAxes.setLogY()
PlotAxes.setWindow()
PlotAxes.setXDrawing()
PlotAxes.setXtickCallback()
PlotAxes.setYDrawing()
PlotAxes.setYtickCallback()
PlotAxes.ticksize
PlotAxes.xtick
PlotAxes.ytick
PlotContour
PlotCurve
PlotSurface
Point
RegionOfInterest
Text
delay()
isinstanceof()
islistof()
ndctowc()
readfile()
wctondc()
- gr.pygr.mlab Reference
- gr3 Reference
- grm Reference